일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- Eclipse
- Maven
- 이클립스
- date
- 문자열
- table
- list
- 정규식
- Visual Studio Code
- Button
- 자바스크립트
- javascript
- input
- string
- CMD
- js
- Array
- 자바
- 인텔리제이
- 배열
- 테이블
- vscode
- ArrayList
- json
- 이탈리아
- Java
- windows
- IntelliJ
- CSS
- html
- Today
- Total
어제 오늘 내일
[Java] 파일 생성하는 3가지 방법 (File, FileOutputStream, Files) 본문
지난 번에는 Java API를 이용하여,
텍스트 파일을 읽는 방법을 알아보았습니다.
[Java] 텍스트 파일 읽기 ( FileReader, BufferedReader, Scanner, Files )
[Java] 텍스트 파일 읽기 ( FileReader, BufferedReader, Scanner, Files )
Java에서는 여러 가지 방법으로 텍스트 파일의 내용을 읽을 수 있습니다. 이번 글에서는 Java에서 텍스트 파일을 읽는 방법을 소개합니다. FileReader BufferedReader Scanner Files 먼저, 아래의 내용이 담긴
hianna.tistory.com
이번에는, 파일을 생성하는 3가지 방법을 알아보도록 하겠습니다.
- java.io.File
- java.io.FileOutputStream
- java.nio.file.Files
1. java.io.File
public boolean createNewFile() throws IOException
File 클래스의 createNewFile() 메소드를 이용하여, 새로운 파일 및 디렉토리를 생성할 수 있습니다.
이 메소드는,
파일을 성공적으로 생성하면, true를 리턴하고,
기존에 이미 동일한 이름의 파일이 있을 경우에는 false를 리턴합니다.
createNewFile() 로 파일 생성 하기 - 코드
import java.io.File;
import java.io.IOException;
public class CreateFile {
public static void main(String[] args) {
File file = new File("d:\\example\\file.txt");
try {
if (file.createNewFile()) {
System.out.println("File created");
} else {
System.out.println("File already exists");
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
createNewFile() 로 파일 생성 하기 - 결과 1
java.io.IOException: 지정된 경로를 찾을 수 없습니다
at java.base/java.io.WinNTFileSystem.createFileExclusively(Native Method)
at java.base/java.io.File.createNewFile(File.java:1026)
at CreateFile.main(CreateFile.java:10)
위 결과는, 코드에서 지정한 example 디렉토리가 없을 경우 발생합니다.
createNewFile() 메소드는 파일만 새로 생성합니다.
만약, 지정한 디렉토리가 존재하지 않으면 IOException을 발생시킵니다.
createNewFile() 로 파일 생성 하기 - 결과 2
File created
example 디렉토리를 생성하고,
처음 위 코드를 실행하면, example 디렉토리에 비어있는 file.txt 파일이 생성되고,
createNewFile() 메소드는 true를 리턴합니다.
createNewFile() 로 파일 생성 하기 - 결과 3
File already exists
두번째로, 위 코드를 실행하면,
기존에 만들어진 file.txt 파일이 존재하기 때문에
위 코드는 파일을 새로 생성하지 않고, false를 리턴합니다.
2. java.io.FileOutputStream
public FileOutputStream(String name) throws FileNotFoundException
public FileOutputStream(String name, boolean append) throws FileNotFoundException
public FileOutputStream(File file) throws FileNotFoundException
public FileOutputStream(File file, boolean append) throws FileNotFoundException
public FileOutputStream(FileDescriptor fdObj)
FileOutputStream의 생성자를 이용해서 파일을 생성할 수 있습니다.
첫번째 파라미터로,
생성할 파일의 이름 문자열, File 객체, FileDescriptor 객체를 전달합니다.
두번째 파라미터(append)로,
만약 생성하려는 파일이 존재할 경우,
기존 파일을 열어서 그 뒤에 이어서 파일을 작성할지
기존 파일을 덮어쓰고 새로 파일을 생성할지를 지정해 줍니다.
만약, append 값을 true이면,
기존 파일이 있을 경우, 기존 파일을 열어서 그 파일에 내용을 이어붙입니다.
그러나, append 값이 false인 경우, 기존 파일을 덮어쓰기 되므로 주의해야 합니다.
두번째 파라미터(append)를 입력하지 않을 경우, 기본값은 false입니다.
FileOutputStream으로 파일 생성 하기 - 코드
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
public class CreateFile {
public static void main(String[] args) {
File file = new File("d:\\example\\file.txt");
try {
FileOutputStream fileOutputStream = new FileOutputStream(file, true);
} catch (FileNotFoundException e) {
e.printStackTrace();
}
}
}
FileOutputStream으로 파일 생성 하기 - 결과 1
java.io.FileNotFoundException: d:\example\file.txt (지정된 경로를 찾을 수 없습니다)
at java.base/java.io.FileOutputStream.open0(Native Method)
at java.base/java.io.FileOutputStream.open(FileOutputStream.java:298)
at java.base/java.io.FileOutputStream.<init>(FileOutputStream.java:237)
at CreateFile.main(CreateFile.java:11)
디렉토리가 존재하지 않을 경우, 위와 같이 FileNotFoundException을 발생시킵니다.
FileOutputStream으로 파일 생성 하기 - 결과 2
디렉토리를 만들어주고, 위 코드를 실행하면
위 그림과 같이 비어있는(0KB) 파일이 정상적으로 생성된 것을 확인 할 수 있습니다.
FileOutputStream으로 파일 생성 하기 - 결과 3
FileOutputStream fileOutputStream = new FileOutputStream(file, true);
만약, 위 코드를 실행하기 전에
이미 d:\example\file.txt 파일이 존재하고,
이 파일에 내용이 있을 경우,
FileOutputStream 생성자의 두번째 파라미터(append)의 값이 true이기 때문에
기존 파일이 유지됩니다.
FileOutputStream으로 파일 생성 하기 - 결과 4
FileOutputStream fileOutputStream = new FileOutputStream(file, false);
FileOutputStream 생성자의 두번째 파라미터(append)를 false로 할 경우,
기존 파일이 지워지고 새로운 빈 파일이 만들어집니다.
3. java.nio.file.Files
public static Path createFile(Path path, FileAttribute<?>... attrs) throws IOException
Java 7이후부터는 Files.createFile() 메소드를 이용해서 파일을 생성할 수 있습니다.
두번째 파라미터인 FileAttribute 객체는 Optional로, 필수가 아닙니다.
Files로 파일 생성 하기 - 코드
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class CreateFile {
public static void main(String[] args) {
Path filePath = Paths.get("d:\\example\\file.txt");
try {
Path newFilePath = Files.createFile(filePath);
System.out.println(newFilePath);
} catch (IOException e) {
e.printStackTrace();
}
}
}
Files로 파일 생성 하기 - 결과 1
java.nio.file.NoSuchFileException: d:\example\file.txt
at java.base/sun.nio.fs.WindowsException.translateToIOException(WindowsException.java:85)
at java.base/sun.nio.fs.WindowsException.rethrowAsIOException(WindowsException.java:103)
at java.base/sun.nio.fs.WindowsException.rethrowAsIOException(WindowsException.java:108)
at java.base/sun.nio.fs.WindowsFileSystemProvider.newByteChannel(WindowsFileSystemProvider.java:235)
at java.base/java.nio.file.Files.newByteChannel(Files.java:371)
at java.base/java.nio.file.Files.createFile(Files.java:648)
at CreateFile.main(CreateFile.java:12)
파일을 생성하려는 디렉토리가 존재하지 않을 경우
(여기서는 d:\example 디렉토리가 없을 경우)
NoSuchFileException이 발생합니다.
Files로 파일 생성 하기 - 결과 2
d:\example\file.txt
이번에는 d:\example 디렉토리를 생성한 후 위 코드를 실행시켰습니다.
정상적으로 빈 파일이 생성되고, Files.createFile() 메소드는 Path 객체를 리턴하였습니다.
Path 객체의 toString()을 이용하여, 생성된 파일의 경로 및 이름을 출력하였습니다.
Files로 파일 생성 하기 - 결과 3
java.nio.file.FileAlreadyExistsException: d:\example\file.txt
at java.base/sun.nio.fs.WindowsException.translateToIOException(WindowsException.java:87)
at java.base/sun.nio.fs.WindowsException.rethrowAsIOException(WindowsException.java:103)
at java.base/sun.nio.fs.WindowsException.rethrowAsIOException(WindowsException.java:108)
at java.base/sun.nio.fs.WindowsFileSystemProvider.newByteChannel(WindowsFileSystemProvider.java:235)
at java.base/java.nio.file.Files.newByteChannel(Files.java:371)
at java.base/java.nio.file.Files.createFile(Files.java:648)
at CreateFile.main(CreateFile.java:12)
생성하려는 파일이 이미 있을 경우,
FileAlreadyExistException이 발생합니다.
Java API를 이용하여 파일을 생성하는 3가지 방법을 알아보았습니다.
다음번에는 생성된 파일에 텍스트를 입력하는 방법을 알아보도록 하겠습니다.
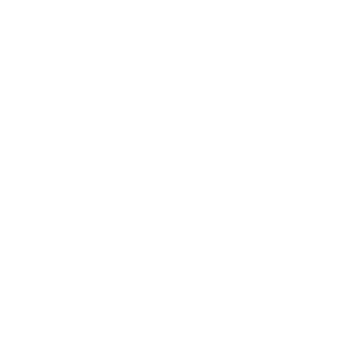
'IT > Java' 카테고리의 다른 글
[Java] 파일에 텍스트 쓰기 (1) | 2021.05.29 |
---|---|
[Java] 파일, 디렉토리 존재 여부 확인하기 (0) | 2021.05.29 |
[Java] 텍스트 파일 읽기 ( FileReader, BufferedReader, Scanner, Files ) (2) | 2021.05.29 |
[Java] 키보드로 사용자 입력받는 2가지 방법 (BufferedReader, Scanner) (0) | 2021.05.24 |
[Java] List에서 공백, null 제거하기 (0) | 2021.05.23 |